fast-xml-parserをインストール
npm install fast-xml-parser
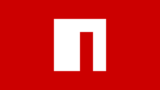
fast-xml-parser
ValidateXML,ParseXML,BuildXMLwithoutC/C++basedlibraries.Latestversion:4.4.0,lastpublished:2monthsago.Startusingfast-xml-parserinyourprojectbyrunning`npmifast-xm...
XMLをパースして、attributeを参照して、最後にXMLに差し戻して保存する感じの処理です。
const { XMLParser, XMLBuilder, XMLValidator } = require('fast-xml-parser');
const options = {
ignoreAttributes: false,
format: true,
};
const parser = new XMLParser(options);
const xmlSample = `
<?xml version="1.0" encoding="utf-8"?>
<root>
<item id="fuga">aaa</item>
<item id="piyo">bbb</item>
</root>`;
const filePath = path.join(__dirname, 'hoge.xml');
const xmlStr = fs.readFileSync(filePath, 'utf-8').toString();
const xmlObj = parser.parse(xmlStr);
for (let i = 0; i < xmlObj.root.item.length; i++) {
const item = xmlObj.root.item[i];
const idKey = '@_id';
const textKey = '#text';
const id = item[idKey];
console.log(id);
// => fuga
const text = item[textKey];
console.log(text);
// => aaa
}
const xmlContent = builder.build(xmlObj);
fs.writeFileSync(path + 'piyo.xml', xmlContent, function (err) {
if (err) return log.error(err);
});
ignoreAttributesは未設定の場合はtrueとなるため、オプション未指定の場合はattributeがパースされません。
また、出力時にのみ影響しますがformat: trueとしておけばelement毎に改行されて表示されます。こちらはデフォルトでfalseなので1行で出力されます。
const options = {
ignoreAttributes: false,
format: true,
};
const idKey = '@_id';
const textKey = '#text';
まあfast-xml-parserはattributeの指定にはprefixとして”@_”が必要です。
またelementの値は”#text”で取得できます。
オブジェクトにattributeを足す場合も
item[“@_hoge”] のようにprefix付きで値を足すとbuildすればattributeとして出力できます。
コメント