install
cntl+D exit()
venvを用いて仮想化環境の作成
python -m venv py3env
仮想化環境の有効化
python_envに移動してから以下を実行する。
source py3env/bin/activate
基礎知識
: 条件文末につける
if age >= 20:
print("あなたの年齢は20歳以上ですね!")
テキストの読み込み
第2引数にreadならr、writeならw、両方ならaを指定する。
f = open("data.txt","r")
if文 pass
pythonでは中身が省略できない。
なので条件分岐をしたが、何も実行させたくない時にpassを使う。
if condition:
pass
else:
pass
continue / pass
passは何もしない。処理を止めもしないし、なにか処理をするわけでもない。
一方、ifやfor文で使われるcontinueは以降の処理を行わず、次のステップに移るという動作になる。なのでcontinue以下に処理を書いても読まれない。
None
nilでもnillでもnullでもなく、None.
しかも先頭は大文字。
JSでいうundefinedもnullもNoneで一括り。
if object と書かずに、
if object is not Noneと書きましょう。
Comparisons to singletons like None should always be done with is
or is not, never the equality operators.
Also, beware of writing if x
when you really mean if x is not None
— e.g. when testing whether a variable or argument that defaults to None was set to some other value. The other value might have a type (such as a container) that could be false in a boolean context!
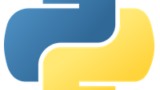
.format() 式展開
{}を.format()の引数で順に置き換える。
hoge = 55
fuga = 22
"hogehoge{}hoge{}hoge".format(fuga,hoge)
=> hogehoge22hoge55hoge
.join()
stringに対してjoin(array)と指定する。
stringを間に挟んで結合される。rubyとは逆なので気持ち悪い。
hoge = "|".join(arr)
index付きのfor文
forで回せるオブジェクトをenumerateすると、forの値を2種類持てる。
1つ目が要素の番号で、2つ目が要素の値になる。
for i,r in enumerate(root):
iがindex、rがrootのvalue
switch文
存在しない。if文の列挙で対応する。
XML解析
pythonは標準モジュールでxml解析が可能。
import xml.etree.ElementTree as ETがそれ。
import xml.etree.ElementTree as ET
root = ET.fromstring(xml_string)
print(root)
print(root.tag,root.attrib)
print(root.tag)
for child in root:
print(child.tag, child.attrib)
XMLの構造
<HOGE_result>
<result name="hoge"/>
<result name="hoge"/>
<result name="hoge"/>
<result name="hoge"/>
<result name="hoge"/>
<FUGA_value>
<value kana="ホゲ"/>
<value kana="ホゲ"/>
<value kana="ホゲ"/>
</FUGA_value>
</HOGE_result>
ノードがネストされていく構造。
ノード.tagでノード名称が(上の例だとresultやFUGA_value)
ノード.attribで配下の属性情報が出力される。
({‘name’: ‘hoge’}や{‘kana’: ‘ホゲ’})
ネストしている場合に直接属性情報を持たないものはHOGE_result.attribは{}を返す。
rootに対してchildを指定することで子要素を全て抜粋できる。
for child in root:
print(child.tag,child.attrib)
rootはタグと属性の辞書を持ち、イテレート可能な子ノードを持つ。
.iter()
タグ名を引数にもち、タグ名のノードを取得する。
深い階層でも取得できる。
for child in root.iter('value'):
.findall()
タグ名を引数にもち、タグ名のノードを取得する。
iter()と異なり、直下の子ノードのみが対象。
for result in root.findall('result'):
etreeクラス
.get()
nodeのattributeを.attribで取り出した際にはetreeクラスとなるため、stringに対するメソッドが使えない。{hoge: fuga, hoge2: fuga2,,,}
attribute内のvalueを取り出すためにr.get(“hoge”)と指定するとfugaを引ける。
root = read_xml(path)
for i,r in enumerate(root):
r.get("contextRef")
requests
pythonライブラリ。
requests.get(‘URL’) で GET リクエストができる.
レスポンスに対して .text とすることで, レスポンスボディをテキスト形式で取得できる.
re
正規表現オブジェクト
re.compile()で正規表現オブジェクトへ変換できる。
文字列の前にrをつけるとエスケープシーケンスを使わずにかける。
以下の例ではrはあってもなくても変わらないが、\\と打たなくて済むので楽。
import re
pattern = r"四半期"
repattern = re.compile(pattern)
match()
文頭から文字列一致を検索。
全文検索はsearchを使う。
import re
m = re.match(pattern, string):
print(m)
=> <re.Match object; span=(2, 5), match='四半期'>
group() | 正規表現にマッチした文字列を返す。 |
start() | マッチの開始位置を返す。 |
end() | マッチの終了位置を返す。 |
span() | マッチの位置 (start, end) を含むタプルを返す。 |
コメント